C#项目,一般有默认的配置文件,Web项目下是web.config,应用程序项目下是app.config。
我们通常可以通过ConfigurationManager类,它直接读取AppSetting节中的配置项。
但如果用ConfigurationManager的AppSettings来读取配置项呢,那么对应的配置文件名必须是app.config,但有时候我们需要自定义配置文件,那么如何处理呢?
1、创建独立的配置文件,配置文件格式建议为utf-8编码,文件扩展名为.config
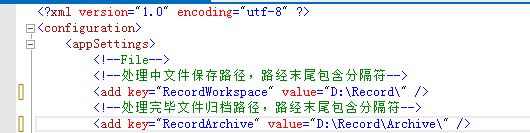
2、编写ConfigHelper类文件
class ConfigHelper
{
private static Configuration _config;
static ConfigHelper()
{
string configPath = GetAssemblyPath() + Path.DirectorySeparatorChar + "*********.config";
ExeConfigurationFileMap map = new ExeConfigurationFileMap();
map.ExeConfigFilename = configPath;
_config = ConfigurationManager.OpenMappedExeConfiguration(map, ConfigurationUserLevel.None);
}
/// <summary>
/// 获取Assemble所在路径
/// </summary>
/// <returns>Assemble所在路径,路径末尾不含分隔符</returns>
public static String GetAssemblyPath()
{
return Path.GetDirectoryName(Assembly.GetExecutingAssembly().Location);
}
public static void SetAppSeting(string key, string value)
{
if (key.Length == 0)
{
throw new ApplicationException("配置参数名称不能为空");
}
try
{
_config.AppSettings.Settings[key].Value = value;
_config.Save(ConfigurationSaveMode.Modified);
}
catch (ConfigurationException ex)
{
throw new ApplicationException("配置参数[" + key + "]更新失败,请检查对应Config文件中appSettings节", ex);
}
}
/// <summary>
/// 在Config文件中,根据配置参数名称查询appSettings节,获取对应配置参数值
/// </summary>
/// <param name="key">配置参数名称</param>
/// <returns>配置参数值</returns>
/// <exception cref="ApplicationException">无法获取,抛出相应异常</exception>
public static string GetAppSetting(string key)
{
string ret = null;
if (key.Length == 0)
{
throw new ApplicationException("配置参数名称不能为空");
}
try
{
ret = _config.AppSettings.Settings[key].Value;
}
catch (ConfigurationException ex)
{
throw new ApplicationException("配置参数[" + key + "]获取失败,请检查对应Config文件中appSettings节", ex);
}
return ret;
}
/// <summary>
/// 在Config文件中,根据配置参数名称查询connectionStrings节,获取对应配置参数值
/// </summary>
/// <param name="key">配置参数名称</param>
/// <returns>配置参数值</returns>
/// <exception cref="ApplicationException">无法获取,抛出相应异常</exception>
public static string GetConnectionSetting(string key)
{
string ret = null;
if (key.Length == 0)
{
throw new ApplicationException("配置参数名称不能为空");
}
try
{
ret = _config.ConnectionStrings.ConnectionStrings[key].ConnectionString;
}
catch (ConfigurationException ex)
{
throw new ApplicationException("数据库链接字符串" + key + "获取失败,请检查Config文件中connectionStrings节", ex);
}
return ret;
}
}
3、在需要操作config文件内容时,可直接使用
m_RecordWorkspace = ConfigHelper.GetAppSetting("RecordWorkspace");
m_RecordArchive = ConfigHelper.GetAppSetting("RecordArchive");
ConfigurationManager是针对非Web类项目的,相应的Web项目通常其配置文件为web.config,对应的WebConfigurationManager,其也有对应的方法,可以操作自定义配置文件。